I have been listening to a lot of audio books lately, which I have typically imported from CDs onto my iPhone via iTunes. Most of the Icelandic audio CDs I have listened to are in MP3 format and described in the SMIL markup language, which iTunes has no concept of. The files are named 01.mp3, 02.mp3, ... and so on and are void of any ID3 information, with all the metadata in those .smil files. Anywho, to my frustration once imported into iTunes, neither iTunes nor my iPhone have the good sense of playing the files in the correct order. Why they play them in some arbitrary order other than by file name, I have no idea, and iTunes does not seem to have any way of changing the play order. So I figured I needed some way to join the files into a single MP3 file so iTunes/iPhone couldn't mess up the order. After googling around for products to join MP3 files and mainly finding shareware software that I wasn't willing to pay for, I stumbled up on the fact that since MP3 is a streaming format you can simply concatenate MP3 files using the good old Unix cat command! So I came up with the following one-liner to join the files together (in alphabetic order), which happily runs on my Mac:
find /Volumes/My\ Disc/*.mp3 -exec cat {} >> MyAudioBook.mp3 \;
Where "/Volumes/My\ Disc/" is the Mac CD drive, and MyAudioBook.mp3 is the final output file that can be imported into iTunes.
Anyways, I figured I'd share this one, as I have to admit I was surprised to learn that MP3 files could simply be "cat"ed together :-)
Blog about software development, agile practices, software use, and the latest happenings in the wide world of technology
Saturday, October 10, 2009
Thursday, January 22, 2009
Don't Click, SlickRun!

I recently discovered the Windows utility SlickRun, thanks to a comment from Jim Holmes in this blog entry from Jeff Blankenburg. I have to say that I absolutely love this utility and at this point can't imagine work without it! It has saved me numerous mouse-clicks and tedious search and navigation through Windows menus. If you are unfamiliar with this utility it basically allows you to start almost any application or file on your computer with just a few strokes on your keyboard. For example if I type "[W]-q memlogin" it opens up my IDE (unfortunately BEA Workshop) with the Membership-Login project that I am currently working on. Or if I type "[W]-q wls" it starts my WebLogic Server instance. Or if I type "[W]-q wikip la lakers" it will fire up my default web browser and take me to the Wikipedia entry for The Los Angeles Lakers (where "la lakers" is a dynamic search term). The words "memlogin", "wls" and "wikip" are keywords (SlickRun calls them MagicWords) that I have configured to fire up stuff that I care about. I can type those directly into the SlickRun prompt (see image at the top of this blog entry) which can be placed anywhere on your screen. I have mine auto-hide and then use the "[W]-q" key sequence to bring the SlickRun program into focus ([W] being the Windows Key on my keyboard).
I spent some time creating keywords for pretty much anything I use at work. Here are some of things I have set up:
- Keyword for every Java project I have worked on at my current client.
- Keyword for each Wiki page I maintain at my current client
- Keyword for each website I frequent. Using the "$W$" marker to plug search parameters into URLs that have query strings.
- Keyword for each application I regularly use (MS Office apps, FireFox, XMLSpy, Cygwin, WinMerge, NoteTab, Toad, Putty, SoapUI, JMeter, Eclipse, etc.)
- Keywords for folders I frequently need to access on my computer (My Documents, c:\user_projects, etc). The keywords will open up the appropriate folder in Windows Explorer.
- Keywords for my most frequently used Windows operations. For example to go to standby, to shutdown, to put on the screen saver, or to open up the display configuration (which my docking station keeps getting messed up).
If you are tired of mouse-clicks and menu navigation I suggest you give this tool a try. It just might make your workday a little more productive. It can't hurt to give it a try, it is free! Be forewarned though that the product could be a little more polished, especially their setup screen which did some funny things for me, but once you get it all set up it does work very well.
Saturday, January 10, 2009
CodeMash 2009
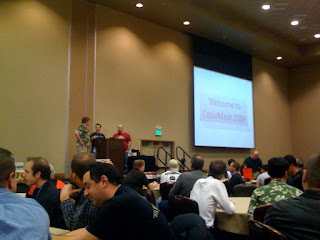
![]() | ![]() |
Below is a sprint through the sessions I attended this year.
Day 0 - PreCompiler
Groovy and Grails 101 w. Chris Judd and Jim Shingler
This was a good overview session on Groovy and Grails. Groovy in the morning, Grails in the afternoon. Chris did a good job of explaining all the things that suck about Java, by showing a Java class and then piece by piece groovy-tizing it down to less than half of its original size. The last lab of the morning involved writing code to read an RSS feed and write the ids and title of all blog postings to a relational database, then pull it back from the db and spit out as an XML document. We did this in about 40 min, and I have to say that even with all my Java experience I doubt that I would have been able to pull the same off with Java in that amount of time. Reading from an http url, extracting info from an XML document, writing to a database, reading from a database and creating an XML document was all very easy in Groovy and required very few lines of code. Here is the code that did it:
import groovy.sql.Sql
//Read the RSS feed
def feed = new URL("http://stanjonsson.blogspot.com/feeds/posts/default").text
//Create a database instance
def sql = Sql.newInstance(/jdbc:derby:C:\temp\blogs;create=true/, "APP",
"APP", "org.apache.derby.jdbc.EmbeddedDriver")
//Delete table if previously created
try {
sql.execute("drop table blogs")
} catch(Exception e){}
//Create table
sql.execute('''create table blogs (
id varchar(200) not null primary key,
title varchar(500)
)''')
//Parse XML and populate the table
def blogs = sql.dataSet("blogs")
feed = new XmlSlurper().parseText(feed)
feed.entry.each {entry ->
blogs.add( id: entry.id.text(), title: entry.title.text() )
}
//Read from table and create Xml
def writer = new StringWriter()
def builder = new groovy.xml.MarkupBuilder(writer)
builder.setDoubleQuotes(true)
builder.feedlist {
sql.eachRow("select * from blogs") { row ->
feedentry(id:row.id) {
title row.title
}
}
}
//Print out XML, and voilà, done!
println writer.toString()
Pretty easy heh? No try-catch blocks, no headaches around setting up and closing database resources, no InputStreams, no importing an external XML parser. And the code even creates the database and table on the fly!!
For those curious the output from running the above is:
<feedlist>
<feedentry id="tag:blogger.com,1999:blog-2241013152284479770.post-720388331940374029">
<title>No Fluff Just Stuff 2008</title>
</feedentry>
<feedentry id="tag:blogger.com,1999:blog-2241013152284479770.post-1855527957885191012">
<title>Career 2.0</title>
</feedentry>
</feedlist>
Which, hehum, proves that I really need to be more active in my blogging.
During the afternoon session we went over the Grails framework, which is highly reminiscent of Rails. Jim showed us how to quickly put together a web site by defining a couple of domain objects and having Grails do its magic by generating the persintance layer and database tables and a nifty front end for CRUD operations on the domain objects. It looked really nice, but started loosing some of its charm once he started mocking with the view GSPs and we came to realize it effectively made any further auto-generation from the domain objects impossible (or you would overwrite your changes). So the lesson learned is to put detailed thought into designing your domain objects up front and save your self some work by having Grails auto-generate your views, controllers and persistence layer (via GORM, a Groovy object relational mapping implementation on top of Hibernate). But know that once you have put some work into customizing your application any changes to your domain objects means you will need to edit your views, controllers and database mappings by hand.
Here are the slides from the PreCompiler.
Day 1
Introducing iPhone SDK - Chris Adamson
This was a session had been looking forward to a lot. I have been a trusty iPhone 3G owner since it was released last July (I even stood in line before stores opened the day it was released!) and have been eyeing getting into iPhone development. The session provided a good overview of what it takes to get into iPhone development and walked through the implementation of a simple application. Unfortunately the starting cost is somewhat high; get a Mac, learn Objective-C, pay for a development license, deal with Apple's registration process, write your app and wait for up to month to get it approved. Objective-C is a Smalltalk inspired C like language dating back to 1986. I must admit that manual memory management and pointers aren't exactly things I have missed for the past 10 years or so. So delving into Objective-C is something I'd do with some hesitation. Actually, Chris Judd's iPhone with Grails presentation on Day 2 got me thinking I might start by doing an iPhone web application rather than native application.
Slides from the presentation.
Three tips to improve your dev process - Jim Holmes
Former Quick Solutions colleague Jim Holmes had an interesting talk on things we can do to improve our software development processes. He discussed the importance of daily standups and retrospectives, and provided tips to improve estimation. The session was mainly memorable for the somewhat heated discussion that materialized between Jim and a few of his audience members, headed by Mary Poppendieck, who as Jim put it "lead him out on a branch and then proceeded to saw it off".
Slides to be posted at FrazzledDad.com.
Erlang: The Basics - Kevin Smith
Very interesting session and by far the one that made my eyes spin the most. As Kevin put it "Erlang Is Weird". If you can not imagine life without for-loops, while-loops or strings, then Erlang is not for you! I enjoyed getting familiar with the language, but to be honest, don't see myself as a hard core Erlang fanboy anytime soon. But due Erlang's strength in dealing with concurrency I imagine the language will continue to prosper in a world where 256 core CPUs will soon be a reality.
Since there are no loops in Erlang, recursion is the name of the game. Here is a simple Erlang code to print out the contents of a list:
print([H|T]) ->
io:format(“~p~n”, [H]),
print(T);
print([]) ->
ok.
I found it interesting that the language has three (!) line terminators (, ; and .), when most of the new generation languages (Ruby, Python, Groovy, Scala, etc.) have gone the route of dropping terminators.
Slides here.
iPhone development with Grails - Chris Judd
Another session I was looking forward to. Chris walked us through the pros and cons of doing native vs. web application development for the iPhone. The pros of native development being:
- Access to native features like GPS, accelerometer and camera.
- Offline access.
- Performance.
- The glamour and marketing associated with having your app in the AppStore.
The pros of web app development being:
- Don't have to learn Objective-C. You can develop with whatever technology you are most comfortable with.
- Deployment under your control.
- Don't have to get a development license.
- Don't have to bury yourself in a mountain of legal agreements.
- The process is on your timeline and not Apple's
Chris then walked us through developing an iPhone web application using Grails with a custom iPhone plugin that he wrote for the iUI JavaScript framework, which allows you to customize the look and feel of your web app to rival that of a native application.
I have been brewing an idea for an application that I would like to write for my phone. Since the benefits of writing it as a native application are limited and clients will require access to a centralized database, I am making it my mission to write it as a Grails web app in 2009. I even went ahead and registered the domain goodweatherlocator.com just now, which should give you some idea about what I am planning to build.
Slides from the session here.
Sexier Software With Flex - James Ward
This was an overview session on how to build applications using Flex. It showed some pretty sexy Flex applications and then walked us through the creation of a basic Flex app using MXML and ActionScript.
I have never been very interested in RIA development, but figured I would at least dip my toe in the pool.
If you are unfamiliar with Flex, check out the tour at http://flex.org/tour.
Day 2
Practices of an Agile Developer - Venkat Subramaniam
As in his keynote a day earlier Venkat was excellent in this no frills Agile session. He provided many good tips on how to get into Agile and how to practice Agile software development, along with some gold nuggets on how to improve yourself as a software developer:
- The most important thing in adopting Agile is attitude.
- Criticize ideas, not people. Take pride in arriving at a solution rather than proving whose idea was best.
- Learn everything about something and something about everything.
- It is very hard to learn if don't learn continuously. It is incredibly easy if you do it all the time.
- Spend 30 min a day reading something unrelated to your current job.
- Present details and let your customers decide. Developers, managers or business analysts shouldn't make business critical decision - let the business owners make those.
- Run your unit tests on all platforms you plan to support.
- Use standup meetings to keep the team on the same page.
No slides, but Practices of an Agile Developer by Venkat and Andy Hunt a recommended reading. A colleague of mine won a copy of it at the closing ceremony and from my 5 min of browsing through it looked very promising.
Cool Stuff With computer Vision - Scott Preston
Scott showed us his robot Feynman and how he sees the world. Apparently robots are really into Jessica Alba because much of the presentation focused on a beach photo of her, seen through various image filters. When he showed us how his bot can discover and zoom in on her breasts, I noticed the lone female in the room moved uncomfortably in her seat.
All in all this was an entertaining session that gets a 10 on the geek scale.
Slides and photos of Jessica's top here.
Spring 3.0 MVC - Ken Sipe
A nice overview of Spring MVC (mainly 2.5 features) and all the benefits it provides over older MVC frameworks like Struts. Takes "convention over configuration" to the max and greatly reduces the amount of Java code needed for a typical Web application. Or as Ken put it, "The next best thing to Grails"!
Slides to be posted here.
Cloud Computing with .Net - Wesley Faler
The last session of the conference. I figured I couldn't go home without seeing at least one .Net related presentation. Wes walked us through the Cloud Computing concept and how it differs from web farms, grids and clusters. The nuts and bolts of it is essentially computing resources on demand. He got me all excited when describing Amazon's EC2 service and the fact that you can use it with numerous different programming languages. But once he gave us some samples of the pricing, my excitement withered away.
Fancy slides here.
This wraps up my CodeMash 2009 experience. As in previous years I had a lot of fun and will definitely be looking to coming back again. From what I've heard CodeMash 2010 will be even bigger and better.
Subscribe to:
Posts (Atom)